What is Tokenizer in Python? Understanding Tokenization in Natural Language Processing
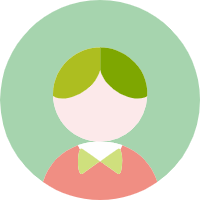
Tokenization is a preprocessing step in natural language processing (NLP) that splits a text into a series of tokens, which are usually words or other grammatical units. Tokenization is essential for many NLP tasks, such as sentiment analysis, machine learning, and text categorization. In this article, we will explore what tokenizer is in Python, how it works, and its applications in NLP.
What is a Tokenizer?
A tokenizer is a function or library that takes a text as input and returns a list of tokens. These tokens can be words, phrases, or other lexical units that are important for NLP tasks. Tokenization is often performed by using different methods, such as word boundary detection, punctuation removal, and stemming. Tokenizers in Python can be implemented using various methods, such as regular expressions, NLP libraries, or custom algorithms.
How Tokenization Works in Python
In Python, tokenization can be achieved using various methods. Here, we will explore some popular methods and their implementation in the Python programming language.
1. Using Regular Expressions (regex)
Regular expressions are powerful tools for matching and extracting patterns from text data. In Python, we can use regex to perform tokenization by matching words and other lexical units. For example:
```python
import re
text = "Hello, I am an AI language model."
tokens = re.findall(r'\b\w+\b', text)
print(tokens)
```
2. Using NLP Libraries, such as NLTK and SpaCy
NLP libraries, such as NLTK and SpaCy, provide pre-built tokenizers and other NLP functions. These libraries usually provide more sophisticated tokenization methods and support for various NLP tasks. For example, using the NLTK library:
```python
import nltk
text = "Hello, I am an AI language model."
tokens = nltk.word_tokenize(text)
print(tokens)
```
3. Using Custom Algorithms
Custom tokenization algorithms can be created using various techniques, such as Trie trees, pos-tagging, and stemming. These algorithms may be more tailored to specific NLP tasks and require more manual effort to implement.
Applications of Tokenization in Natural Language Processing
Tokenization is a crucial step in various NLP tasks, such as:
- Sentiment analysis: Tokenization helps in splitting the text into words or phrases, which can be analyzed for their sentiment, such as positive, negative, or neutral.
- Machine learning: Tokenization is used in preprocessing data for machine learning models, such as text classification, word embeddings, and natural language generation.
- Text categorization: Tokenization helps in splitting the text into categories, such as news articles, social media posts, or customer reviews.
Tokenization is a critical preprocessing step in natural language processing, which helps in splitting text data into useful tokens or words. In Python, tokenization can be achieved using various methods, such as regular expressions, NLP libraries, or custom algorithms. Understanding tokenization and its applications in NLP is essential for developing effective natural language processing systems and applications.